While working as a developer, you must have heard about architectural patterns. When creating various Android app development solutions, most often, you will come across MVC, MVP & MVVM. You probably know their characteristics, but do you know the differences and when to use them?
If you ask yourself these questions, this article is for you.
MVC – Model-View-Controller
Model-View-Controller (MVC) is an architectural pattern that helps organise the structure of our application. It divides its responsibilities into three layers: Model, View, and Controller.
- Model – Data layer, responsible for managing business logic and supporting network or database API. The Model works with the remote and local data sources to get and save the data. This is where the business logic is handled.
- View – UI layer, responsible for data visualisation from the Model to the user. It manages the way data is presented, including the graphical interface.
- Controller – A logical layer that integrates the View and Model layers. The Controller’s job is to take over user input and determine what to do with it.
You can very quickly see how the individual components communicate in the graphic below:
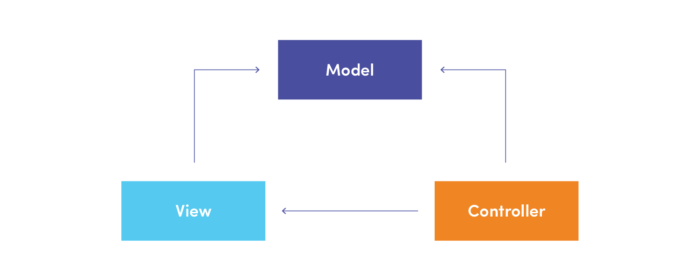
How it works?
Several MVC variants have emerged over the years, but I will mention the two most popular ones here: Passive model and Active model.
Passive Model
In this version of MVC, the Controller is the only class that manipulates the Model. To illustrate this process well, I will use the graphic below:
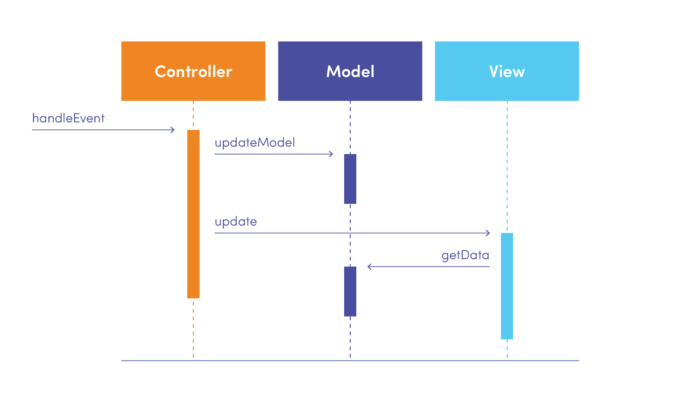
- The Controller responds to the user’s actions and contacts the Model.
- When the Model is changed, the Controller tells the View to update its data.
- The View fetches the updated data from the Model and displays it to the user.
Active Model
In this version of MVC, other classes besides the Controller manipulate the Model.
In this case, the Observer pattern is used, and the View is registered as a Model observer. Thanks to this, the View will be constantly updated when the Model changes.
Advantages
- The MVC pattern greatly supports the separation problem. It increases the testability of the code and facilitates its extension, allowing for easy implementation of new functions.
- The Model class has no reference to the Android system classes, making it very easy to unit test.
- The controller does not extend or implement any Android classes. It makes unit testing possible.
Disadvantages
- The View relates to both the Controller and the Model.
- The View’s dependence on the Model mainly causes trouble in advanced Views. Why? If the Model’s role is to provide raw data, then the View will take over the handling of the user interface logic.
On the other hand, if the Model will display data prepared directly for display, we will get Models that support both business model and UI logic. - Active implementation of the Model increases the number of classes and methods exponentially because observers should be needed for each data type.
- When a View depends on both the Controller and Model, changes to the UI logic may require updates/changes to several classes, thus reducing the flexibility of the design pattern.
- The View’s dependence on the Model mainly causes trouble in advanced Views. Why? If the Model’s role is to provide raw data, then the View will take over the handling of the user interface logic.
- UI logic handling is not limited to one class. For a new programmer, this is quite a problem, and the chance for the division of responsibilities between Model, View and Controller is very high.
- Over time, especially in applications with anemic models, more and more code begins to be sent to the controllers, making them bloated and brittle.
Summary
The dependence of View on the Model and having logic in View can significantly deteriorate the quality of the code in our application. We can reduce this danger by choosing other patterns, particularly those suggested for mobile applications software development. Read about them below.
MVP – Model-View-Presenter
Model-View-Presenter (MVP) is an architectural pattern that we can use to deal with the weaknesses of the MVC pattern. It provides modularity, testability and a much clearer and easier to maintain codebase.
MVP divides the application structure into the View, Model and Presenter layers:
- Model – Analogically to the MVC pattern.
- View – UI layer, responsible for presenting the data to the user in the manner specified by the Presenter. It can be implemented by Activities, Fragments, or any Common view.
- Presenter – The logic layer that mediates between the View and Model layers. It contacts both the View and Model layers and reacts to actions performed by the user.
How it works?
In MVP, View and Presenter are entirely separate and communicate with each other through abstractions. The Contract interface classes define the relationship between them. Thanks to them, the code is more readable, and the connection between the layers is easy to understand. If you are interested in the implementation details, please read Model-View-Presenter: Android guidelines.
It is worth mentioning that the Presenter cannot have any references to an Android-specific API.
See the picture below for a good overview of the data exchange process between the individual components. For the purposes of this article, this example is simplified:
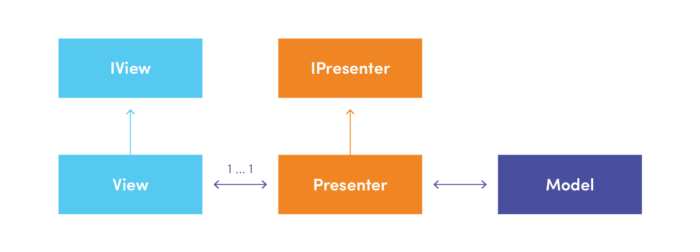
- The user performs the action.
- The Presenter reacts to the user’s action and sends an appropriate request to the Model.
- The model is updated, and new data is sent to the Presenter.
- The Presenter prepares the data for display and sends it to View.
- The View displays the data to the user.
Advantages
- We can easily test the Presenter logic as it is not tied to any Android-specific views and APIs.
- The View and Presenter are entirely separate, which makes mocking a view easy, making unit testing more superficial than then in the MVC
- We only have one class that handles everything related to the presentation of a view – the Presenter.
Disadvantages
- The Presenter, like the Controller, tends to accumulate additional business logic. To solve this problem, break down your code and remember to create classes with only one responsibility.
- While this is a great pattern for an Android app, it can feel overwhelming when developing a small app or prototype.
Summary
Compared to MVC, this pattern is much better. It solves two critical problems of the MVC pattern:
- The View no longer refers to both the Controller and the Model.
- It has only one class that handles everything related to the view’s presentation: the Presenter.
MVVM – Model-View-ViewModel
Model-View-ViewModel (MVVM) is an event-based pattern. Thanks to this, we can react quickly to design patterns and changes. This architectural pattern allows us to separate the UI from business and behaviour logic even more than in the case of MVC or MVP.
- ViewModel – Deals with delivering data from the Model to the View layer and handling user actions. It is worth mentioning that it provides the data streams for View.
- View – The UI layer is responsible for presenting data, system state and current operations in the graphical interface. Apart from that, it initialises and binds ViewModel with View elements (informs ViewModel about user actions).
- Model – Same as MVC – no change.
How it works?
The idea of the MVVM pattern is based primarily on the View layer (Observer pattern) observing the changing data in the ViewModel layer and responding to changes through the data binding mechanism.
The implementation of the MVVM pattern can be achieved in many ways. However, it is worth including the data binding mechanism in it. Thanks to this, the View layer’s logic is minimised, the code becomes more organised, and the testing is easier.

If the MVP pattern meant that the Presenter was directly telling the View what to display, the MVVM ViewModel exposes the event streams to which the Views can be associated. The ViewModel no longer needs to store a reference to the View as it did with the Presenter. It also means that all the interfaces required by the MVP pattern are now unnecessary.
Views also notify the ViewModel of various actions, as seen in the graphic above. Therefore, the MVVM pattern supports two-way data binding between View and ViewModel. The View has a reference to ViewModel, but ViewModel has no information about View.
Advantages
- Unit testing is more straightforward because you’re not addicted to the View. It is sufficient to verify that the observable variables are properly positioned as the Model changes when testing.
- ViewModels are even friendlier to unit testing as they simply expose state and, therefore, can be independently tested without testing how the data will be consumed. In short, there is no dependence on the view.
- Only the View contains a reference to the ViewModel, not the other way around. This solves the tight coupling problem. A single View can reference multiple ViewModels.
- Even for complex Views, we can have different ViewModels in the same hierarchy.
Disadvantages
- Managing ViewModels and their state in complex UI’s is sometimes challenging for the beginners.
Summary
MVVM combines the advantages provided by MVP while using the benefits of data binding and event-based communication. The result is a pattern in which the Model controls as many operations as possible, with high code separation and testability.
MVC, MVP and MVVM: Comparison
MVC | MVP | MVVM | |
---|---|---|---|
Maintenance | hard to maintain | easy to maintain | easy to maintain |
Difficulty | easy to learn | easy to learn | more difficult to learn due to additional functions |
Type of Relation | many-to-one relationship between the Controller and the View | one-to-one relationship between the Presenter and View | many-to-one relationship between the View and ViewModel |
Unit Testing | due to tight coupling, MVC is difficult to unit test | good performance | excellent performance |
Entry Point | Controller | View | View |
References | View doesn’t have reference to the Controller | View has reference to the Presenter | View has reference to the View-Model |
MVC, MVP and MVVM: Summary
Both the MVP pattern and the MVVM pattern fare significantly better than the MVC pattern. Which way you choose really depends on your preferences. However, I hope this article has shown you the key differences between them and will make the choice easier.
Bibliography:
- Cervone, S. (2017) Model-View-Presenter: Android guidelines.
- Dang, A.T. (2020) MVC vs MVP vs MVVM.
- Muntenescu, F. (2016) Android Architecture Patterns Part 1: Model-View-Controller.
- Muntenescu, F. (2016) Android Architecture Patterns Part 2: Model-View-Presenter.
- Muntenescu, F. (2016) Android Architecture Patterns Part 3: Model-View-ViewModel.